The sequence of actions that the script must perform is not complicated, it fully corresponds to the steps that you described in your question:
-
Select one column from the whole sheet (in your case, this is column F, but it can be any)
-
Find a line of text in the selected column (in your case, this is the text “I’m a number”, but it can also be any)
-
If the text is found, then delete the rows with the cells where it was found.
In StarBacic it can be written like this :
Sub deleteRows
delRowsByTextInColumn(ThisComponent.getCurrentController().getActiveSheet(), _
5, "I'm a number")
End Sub
The implementation of the procedure delRowsByTextInColumn() can be of any complexity. For example, the simplest option (without checking the correctness of the input parameters, without handling error situations, is not the most optimal in terms of speed):
Sub delRowsByTextInColumn(oSheet As Variant, nColumn As Long, sText As String)
Dim oColumn As Variant ' Entire column for search '
Dim oSearchDescriptor As Variant
Dim oFound As Variant ' Result of search '
Dim oCells As Variant ' One Part of oFound (subrange) '
Dim oRows As Variant ' All rows of oCells '
Dim i As Long
oColumn = oSheet.getColumns().getByIndex(nColumn) ' 1. One column from the whole sheet '
oSearchDescriptor = oColumn.createSearchDescriptor()
oSearchDescriptor.setSearchString(sText) ' 2a. Set a text to search '
oFound = oColumn.findAll(oSearchDescriptor) ' 2b. Find this text in the selected column '
If Not IsNull(oFound) Then ' 3a. If the text is found... '
For i = oFound.getCount() - 1 To 0 Step -1 ' then for each part of search result '
oCells = oFound.getByIndex(i) ' in backward order '
oRows = oCells.getRows() ' 3b. all rows of subrange '
oRows.removeByIndex(0, oRows.getCount()) ' 3c. remove '
Next i
EndIf
End Sub
However, it should be noted that the use of the available built-in Calc tools is enough to complete your task. Using the Standard Filter allows you to perform the action described by you without writing any macros.
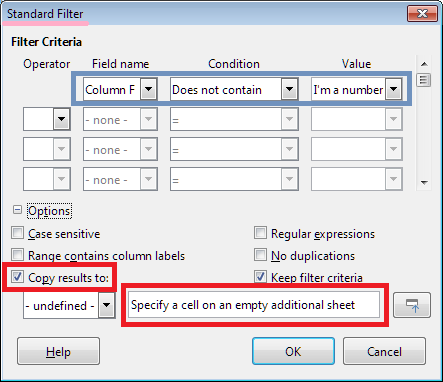