That definitely helps, I missed the LET
function.
But even then, editing large formulas is usually still quite complicated. The editor for formulas just isn’t really great - no code folding, no parenthesis matching, not even a monospaced font.
That makes the rules quickly get complicated. For instance, from real-world business logic of my personal time-keeping:
- The total working time is
end time - start time - unpaid breaks
.
- If the total working time is at least 3 hours in a day, up to 15 minutes can be breaks.
- If the total working time is at least 6 hours in a day, up to 30 minutes can be breaks.
- Paid pauses count as working time, e.g. 1:30 work, 30 minutes break, 1:15 work count as 3 hours of work and 15 minutes of unpaid break.
- In order to avoid messing up the presentation while editing, the value is only calculated if “startTime” and “endTime” are set. “breaks” is allowed to be omitted.
So in python that would be something like (assuming same “1.0 = 24h” units):
def working_hours(start_time, breaks, end_time):
h = 1/24
m = h/60
true_work_time = end_time - start_time - breaks
paid_breaks = min(
breaks,
0.50*h if (true_work_time >= 5.50*h) else
0.25*h if (true_work_time >= 2.25*h) else
0.00*h,
)
return true_work_time + paid_breaks
In Calc format, it would be something like
=LET(
h, 1/24,
m, h/60 + N("Yes, using h here works, but having a real comment syntax would be nice"),
trueWorkTime, endTime - startTime - breaks,
paidBreaks, MIN(
breaks,
IFS(
trueWorkTime >= 5.50*h, 0.50*h,
trueWorkTime >= 2.25*h, 0.25*h,
0.0
)
),
trueWorkTime + paidBreaks
)
… Actually, that looks pretty fine. However, it is rather sabotaged by the formula editor
The use of a not-monospaced font alone does already a lot of damage to getting that right. I guess I might in the future take to editing complicated formulas in Emacs instead 
Anyway… So that’s the first ad-hoc attempt. I got:
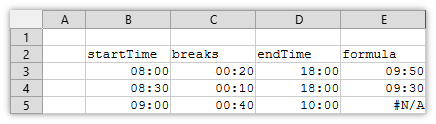
The big difference at that point: With Python I could do simple “print statement debugging”. No such option with Formulas.
Edit. The issue seems to have been with the IFS. I wrongly assumed that it has the behavior of "if last condition has no associated value, assume the condition IS the “else” value. The corrected formula reads
=LET(
h, 1/24,
m, h/60 + N("Yes, using h here works, but having a real comment syntax would be nice"),
trueWorkTime, endTime - startTime - breaks,
paidBreaks, MIN(
breaks,
IFS(
trueWorkTime >= 5.50*h, 0.50*h,
trueWorkTime >= 2.25*h, 0.25*h,
TRUE, 0.0
)
),
trueWorkTime + paidBreaks
)
but that’s exactly a kind of issue that would be much easier to avoid in Python (in this case the equivalent would be a syntax error).
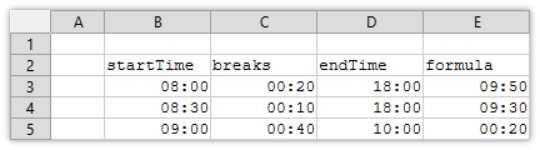