If your data is presented in this form
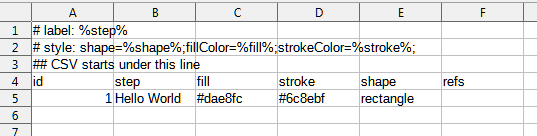
then such a code should solve your problem
Sub ExportToDrawIO()
Dim oCursor As Variant, oData As Variant, oRes As Variant, i As Long
oCursor = ThisComponent.getCurrentController().getActiveSheet().createCursor()
oCursor.gotoEndOfUsedArea(True)
oData = oCursor.getDataArray()
ReDim oRes(LBound(oData) To UBound(oData))
For i = LBound(oData) To UBound(oData)
If Left(oData(i)(0),1) = "#" Then
oRes(i) = Trim(oData(i)(0))
Else
oRes(i) = Join(oData(i), ",")
EndIf
Next i
GlobalScope.BasicLibraries.LoadLibrary("Tools")
SaveDataToFile(ConvertToURL("C:\Output\DrawIO.csv"), oRes)
End Sub
Update. In case the data is on different sheets of the spreadsheet, the code can be like this
Sub ExportToDrawIOFom2Sheets()
Dim oSheets As Variant
Dim oSheet As Variant
Dim oCursor As Variant, oData As Variant, oRes As Variant
Dim i As Long, nRow As Long
oSheets = ThisComponent.getSheets()
oSheet = oSheets.getByName("diagram_config")
oCursor = oSheet.createCursor()
oCursor.gotoEndOfUsedArea(True)
oData = oCursor.getDataArray()
ReDim oRes(LBound(oData) To UBound(oData))
For i = LBound(oData) To UBound(oData)
oRes(i) = Trim(oData(i)(0))
Next i
oSheet = oSheets.getByName("Airstream_Appliances")
oCursor = oSheet.createCursor()
oCursor.gotoEndOfUsedArea(True)
oData = oCursor.getDataArray()
nRow = UBound(oRes) + 1
i = nRow + UBound(oData)
ReDim Preserve oRes(i)
For i = LBound(oData) To UBound(oData)
oRes(nRow + i) = Join(oData(i), ",")
Next i
GlobalScope.BasicLibraries.LoadLibrary("Tools")
SaveDataToFile(ConvertToURL("C:\Output\DrawIO.csv"), oRes)
End Sub