Sorry, I don’t know how to do it with Basic, but this Python code clone all images in source sheet to target sheet.
import uno
import io
import unohelper
from com.sun.star.io import IOException, XOutputStream
from com.sun.star.beans import PropertyValue
CTX = uno.getComponentContext()
SM = CTX.getServiceManager()
class LOShape():
def __init__(self, shape):
self._shape = shape
class OutputStream(unohelper.Base, XOutputStream):
def __init__(self):
self._buffer = b''
self.closed = 0
@property
def buffer(self):
return self._buffer
def closeOutput(self):
self.closed = 1
def writeBytes(self, seq):
if seq.value:
self._buffer = seq.value
def flush(self):
pass
def clone_image(self, doc, to_sheet):
stream = self._shape.GraphicStream
buffer = self.OutputStream()
size, data = stream.readBytes(buffer, stream.available())
stream = SM.createInstanceWithContext('com.sun.star.io.SequenceInputStream', CTX)
stream.initialize((data,))
image = doc.createInstance('com.sun.star.drawing.GraphicObjectShape')
gp = SM.createInstance('com.sun.star.graphic.GraphicProvider')
properties = (PropertyValue(Name='InputStream', Value=stream),)
image.Graphic = gp.queryGraphic(properties)
to_sheet.DrawPage.add(image)
image.Size = self._shape.Size
image.Position = self._shape.Position
return
def clone_shape(self, doc, to_sheet):
for p in self._shape.CustomShapeGeometry:
if p.Name == 'Type':
type_shape = p.Value.title()
service = f'com.sun.star.drawing.{type_shape}Shape'
shape = doc.createInstance(service)
shape.Size = self._shape.Size
shape.Position = self._shape.Position
to_sheet.DrawPage.add(shape)
return
def main():
IMAGE = 'com.sun.star.drawing.GraphicObjectShape'
SHAPE = 'com.sun.star.drawing.CustomShape'
doc = XSCRIPTCONTEXT.getDocument()
source = doc.CurrentController.ActiveSheet
target = doc.Sheets[1]
for shape in source.DrawPage:
s = LOShape(shape)
if shape.ShapeType == IMAGE:
s.clone_image(doc, target)
elif shape.ShapeType == SHAPE:
s.clone_shape(doc, target)
return
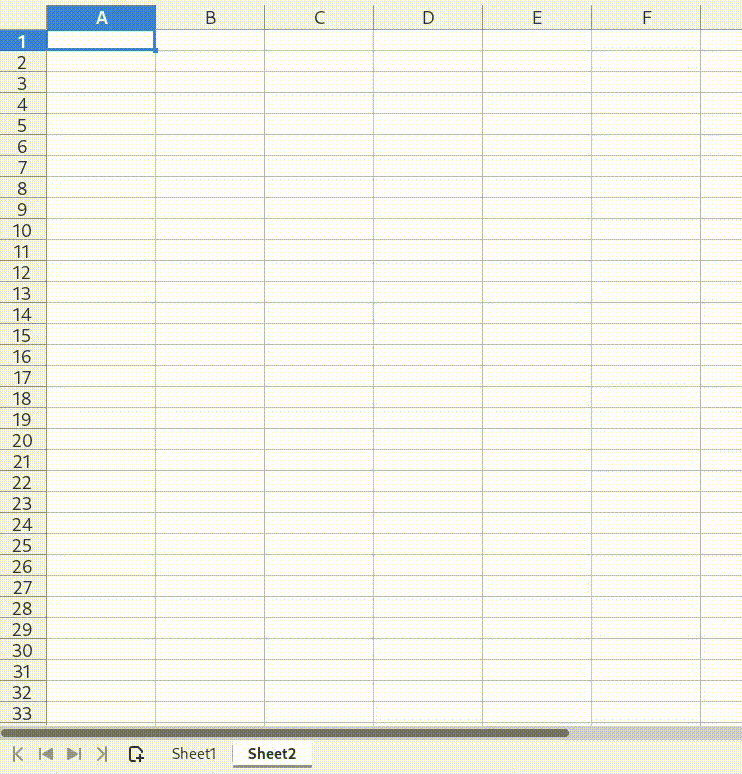